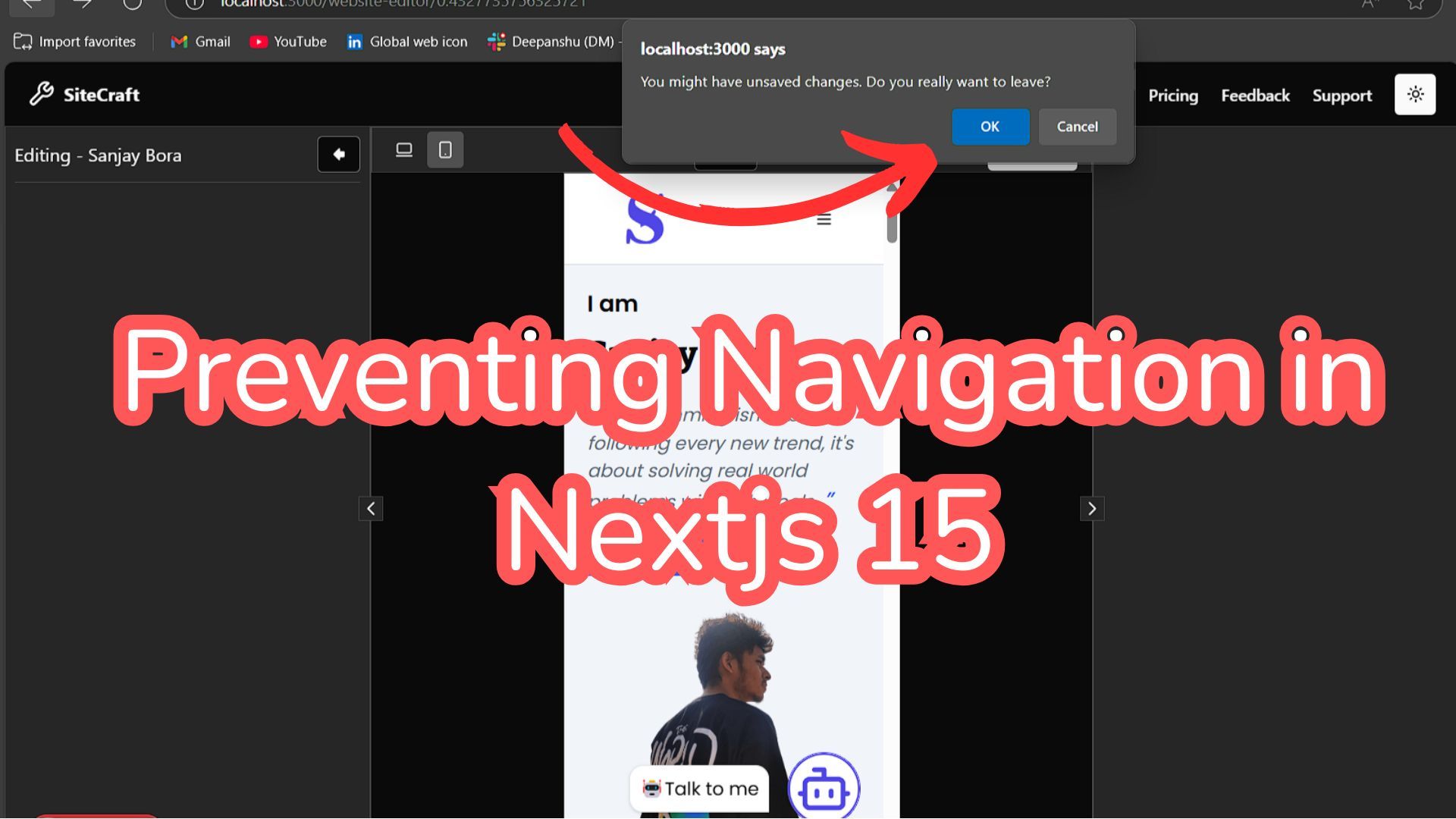
Prevent Navigation in Next.js 15: Here’s How I Solved It
So, I was working on a Next.js 15 project where users had to fill out a form. The last thing I wanted was for them to accidentally click a link, hit the back button, or close the tab and lose all their progress. I needed a way to warn users before they left the page, so I put together this simple solution.
The Problem
I needed to prevent navigation when:
A user clicks a link (<a> tag)
The back button is pressed
The page is reloaded or the tab is closed
Why beforeunload Alone Won’t Work in Next.js
In a traditional web app, adding an event listener for beforeunload would be enough to warn users before they leave. However, Next.js handles routing internally using the client-side router, meaning beforeunload won’t trigger when navigating between pages within the app. That’s why we need additional logic to handle in-app navigation and the back button.
My Solution
After some trial and error, I came up with this useEffect hook:
useEffect(() => {
const interceptNavigation = (event: MouseEvent) => {
const target = event.target as HTMLAnchorElement;
if (target.tagName === "A" && target.href) {
const confirmLeave = window.confirm("You have unsaved changes. Do you really want to leave?");
if (!confirmLeave) {
event.preventDefault(); // Stops navigation
event.stopPropagation();
}
}
};
const handleBeforeUnload = (event: BeforeUnloadEvent) => {
event.preventDefault();
event.returnValue = ""; // Necessary for Chrome
};
const handlePopState = () => {
const confirmLeave = window.confirm("You might have unsaved changes. Do you really want to leave?");
if (!confirmLeave) {
history.pushState(null, "", window.location.href); // Keep them on the page
} else {
window.removeEventListener("popstate", handlePopState); // Allow navigation
}
};
// Prevent immediate back navigation
history.pushState(null, "", window.location.href);
document.addEventListener("click", interceptNavigation, true);
window.addEventListener("beforeunload", handleBeforeUnload);
window.addEventListener("popstate", handlePopState);
return () => {
document.removeEventListener("click", interceptNavigation, true);
window.removeEventListener("beforeunload", handleBeforeUnload);
window.removeEventListener("popstate", handlePopState);
};
}, []);
How It Works
1. Stopping Link Clicks
Listens for clicks on <a> tags.
If a link is clicked, a confirmation popup appears.
If the user cancels, event.preventDefault() stops navigation.
2. Preventing Page Reload & Tab Close
beforeunload fires when the user tries to leave.
event.returnValue = ""; triggers the browser’s warning.
3. Handling the Back Button
When back is pressed, a confirmation appears.
If canceled, history.pushState keeps the user on the page.
4. Initial Back Navigation Prevention
history.pushState ensures the user can’t immediately navigate away.
5. Cleaning Up
Removes all event listeners when the component unmounts.
Will This Work in Other Frameworks?
Yes! While this example is for Next.js 15, the same approach will work in any frontend framework, including React, Vue, and Svelte. The key is handling both internal and external navigation events properly.
The Takeaway
This feature helps prevent users from losing their work by warning them before they navigate away. If you’re building a Next.js 15 app with forms or user inputs, adding this snippet can improve the experience. Let me know if you have a better way to handle this! 🚀